What is Serial and Lot Tracking?
Serial and Lot Tracking are NetSuite inventory management features that enable businesses to track individual items or groups of items by assigning unique serial or lot numbers to each item.
These characteristics are especially important in industries that deal with products that need to be traceable, quality controlled, or comply with regulatory requirements.
Serial and Lot Tracking in NetSuite:
Tracking of Serial Numbers:
As part of serial tracking, each item is given a unique serial number. This enables you to track the movement and history of each item throughout its lifecycle.
Serial Tracking:
Serial tracking involves assigning a unique serial number to each individual item. This allows you to track the movement and history of each specific item throughout its lifecycle.
Serial tracking is commonly used for products that have high value, require warranty tracking, have regulatory requirements, or need to be monitored for quality control.
Key features of Serial Tracking in NetSuite:
Unique Serial Numbers: Each item is assigned a unique serial number, and NetSuite keeps track of which serial numbers correspond to which transactions.
Traceability: By tracking a specific item’s serial number across transactions such as sales, purchases, transfers, and returns, you can easily trace its history and movement.
Warranty and maintenance: Serial tracking is useful for managing individual item warranties and maintenance schedules. This contributes to better customer service and support.
Regulatory Compliance: Certain industries have regulations requiring the tracking of the origin and destination of specific items. Serial tracking aids in ensuring that these regulations are followed.
Lot tracking entails grouping items together under a common lot number, which is commonly used for items produced or received in batches. This is especially important in industries that deal with perishable goods, expiration dates, or quality control concerns.
Lot Tracking in NetSuite has the following features:
Lot Numbers:
Items are assigned to different lots, and each lot is identified by a different lot number. NetSuite keeps track of which items are linked to which lots.
Lot tracking aids in quality control by allowing businesses to trace and recall specific batches of items in the event of quality issues or recalls.
Lot tracking allows you to monitor and manage inventory for products with expiration dates, preventing the sale of expired items.
In NetSuite, both Serial and Lot Tracking features allow you to record transactions involving serialized or allotted items, track their movements, and generate reports for analysis.
These features provide enhanced visibility, accuracy, and compliance in inventory management, catering to the specific needs of businesses across various industries.
Code for Inventory Details
Various transaction records exist within NetSuite, and they can be classified into two groups based on their impact on inventory stock in NetSuite.
Inventory Increase in NetSuite:
- Purchase Order
- Cash Refund
- Positive Inventory Adjustment
Inventory Decrease in NetSuite:
- Item Fulfillment
- Negative Inventory Adjustment
Inventory Increase in NetSuite Code :
When ever we increase the quantity in netsuite we use ‘receiptinventorynumber’ field to set the lot
‘receiptinventorynumber’ type FreeFromText(lotsku)
const rec= record.create({
type: type,
isDynamic: true
})
for (let i = 0; i < rec.line.length; i++) {
rec.selectLine({sublistId: type, line:i});
// set required field
rec.setCurrentSublistValue({
sublistId: string*,
fieldId: string*,
value: number | Date | string | array | boolean*,
ignoreFieldChange: boolean
})
const inventoryDetail= rec.getCurrentSublistSubrecord({ sublistId: 'inventory', fieldId: 'inventorydetail' });
inventoryDetail.selectNewLine({ sublistId: 'inventoryassignment' });
inventoryDetail.setCurrentSublistValue({ sublistId: 'inventoryassignment', fieldId: 'receiptinventorynumber', value: lotsku});
inventoryDetail.setCurrentSublistValue({ sublistId: 'inventoryassignment', fieldId: 'quantity', value: quantity });
inventoryDetail.commitLine({ sublistId: 'inventoryassignment' });
inventoryDetail.commit();
rec.commitLine({ sublistId: type });
}
Inventory Decrease in NetSuite Code :
When ever we decrease the quantity in netsuite we use ‘issueinventorynumber’ field to set the lot
Issueinventorynumber type list(internal_id of pre-existing lot)
const rec= record.create({
type: type,
isDynamic: true
})
for (let i = 0; i < rec.line.length; i++) {
rec.selectLine({sublistId: type, line:i});
// set required field
rec.setCurrentSublistValue({
sublistId: string*,
fieldId: string*,
value: number | Date | string | array | boolean*,
ignoreFieldChange: boolean
})
const inventoryDetail= rec.getCurrentSublistSubrecord({ sublistId: 'inventory', fieldId: 'inventorydetail' });
inventoryDetail.selectNewLine({ sublistId: 'inventoryassignment' });
inventoryDetail.setCurrentSublistValue({ sublistId: 'inventoryassignment', fieldId: 'issueinventorynumber', value: lotInternalId});
inventoryDetail.setCurrentSublistValue({ sublistId: 'inventoryassignment', fieldId: 'quantity', value: quantity });
inventoryDetail.commitLine({ sublistId: 'inventoryassignment' });
inventoryDetail.commit();
rec.commitLine({ sublistId: type });
}
Inventory Details for Inventory Adjustment :
As inventory adjustments can lead to both inventory increases and decreases, we manage inventory adjustments slightly differently by incorporating a conditional check on the quantity.
const rec= record.create({
type: type,
isDynamic: true
})
for (let i = 0; i < rec.line.length; i++) {
rec.selectLine({sublistId: type, line:i});
// set required field
rec.setCurrentSublistValue({
sublistId: string*,
fieldId: string*,
value: number | Date | string | array | boolean*,
ignoreFieldChange: boolean
})
const inventoryDetail= rec.getCurrentSublistSubrecord({ sublistId: 'inventory', fieldId: 'inventory detail' });
inventoryDetail.selectNewLine({ sublistId: 'inventoryassignment' });
if(quantity > 0){
inventoryDetail.setCurrentSublistValue({ sublistId: 'inventoryassignment', fieldId: 'receiptinventorynumber', value:lotNumber });
//if quantity in netsuite use receiptinventorynumber
}else{
inventoryDetail.setCurrentSublistValue({ sublistId: 'inventoryassignment', fieldId: 'issue inventory number', value:lotInternalId });
//if quantity out from netsuite use issueinventorynumber
}
inventoryDetail.setCurrentSublistValue({ sublistId: 'inventoryassignment', fieldId: 'quantity', value: quantity });
inventoryDetail.commitLine({ sublistId: 'inventoryassignment' });
inventoryDetail.commit();
rec.commitLine({ sublistId: type });
}
Summary:
When generating inventory details through a script, it’s important to account for the impact on inventory stock in NetSuite. If the impact is positive, we assign the lot number SKU to the ‘receiptinventorynumber’ field. Conversely, if the impact is negative, we assign the lot number internal ID to the ‘issueinventorynumber’ field.
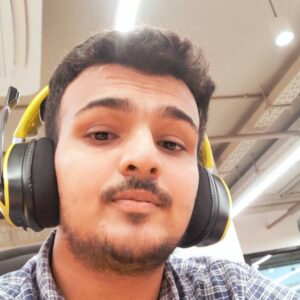
About the Author
Muhammad Sheheryar Vohra
Software Engineer - Folio3
Sheheryar Vohra is a software engineer having experience working with NetSuite. He loves reading books, watching movies and writing blogs